Strange Coincidence
Programming Snapshot – Python Gambling
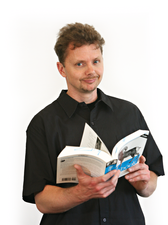
Can 10 heads in a row really occur in a coin toss? Or, can the lucky numbers in the lottery be 1, 2, 3, 4, 5, 6? We investigate the law of large numbers.
If you follow the stock market, you are likely to hear people boasting that their buy and sell strategies allegedly only generate profits and never losses. Anyone who has read A Random Walk down Wall Street [1] knows that wild stock market speculation only leads to player bankruptcy in the long term. But what about the few fund managers who have managed their deposits for 20 years in such a way that they actually score profits year after year?
According to A Random Walk down Wall Street, even in a coin toss competition with enough players, there would be a handful of "masterly" throwers who, to the sheer amazement of all other players, seemingly throw heads 20 times in a row without tails ever showing up. But how likely is that in reality?
Heads or Tails?
It is easy to calculate that the probability of the same side of the coin turning up twice in succession is 0.5x0.5 (i.e., 0.25), since the probability of a specific side showing once is 0.5 and the likelihood of two independent events coinciding works out to be the multiplication of the two individual probabilities. The odds on a hat trick (three successes in a row) are therefore 0.5^3=0.125, and a series of 20 is quite unlikely at 0.5^20=0.000000954. But still, if a program only tries often enough, it will happen sometime, and this is what I am testing for today. The short Python coin_throw()
generator in Listing 1 [2] simulates coin tosses with a 50 percent probability of heads or tails coming up.
Listing 1
cointhrow
01 #!/usr/bin/env python3 02 import random 03 04 def coin_throw(): 05 sides=('heads', 'tails') 06 07 while True: 08 yield sides[random.randint(0, 1)] 09 10 def experiment(): 11 run = 1 12 max_run = 0 13 prev = "" 14 count = 0 15 16 for side in coin_throw(): 17 count += 1 18 19 if prev == side: 20 run += 1 21 if run > max_run: 22 max_run = run 23 print("max_run: %d %s (%d)" % 24 (max_run, side, count)) 25 else: 26 prev = side 27 run = 1 28 29 experiment()
Listing 1 is divided into the coin_throw()
coin toss generator and the experiment evaluation in the experiment()
function. In a for
loop as embedded in line 16, it looks like coin_throw()
would return a long list of result strings consisting of heads
or tails
, but in reality, the function is a dynamic generator that returns a new value whenever an iteration pump like the for
loop asks if there is more coming.
In the Generator House
How does the generator, whose output is shown in Figure 1, work? The key is the yield
command in line 8 of Listing 1. Python interrupts the execution of the current function for a yield
, remembers its internal state, and returns the string (heads
or tails
) to the calling program in line 8. The randint(0,1)
method returns the integer value
or 1
with 50 percent probability, and the script picks one of the two entries in the sides
tuple.
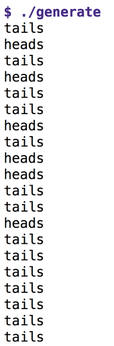
The next time the coin_throw()
function is called, the program flow returns to the previous location within the function to continue where yield
left off. In this case, this is the endless loop with while True
in line 7, whose condition is still true, whereupon another yield
command again returns a random value. Thus the generator produces an endless sequence of values in coin_throw()
and always performs a new coin toss if the for
loop in the main program wants more.
The experiment()
function's remaining code stores the number of identical coin toss outcomes in sequence so far in the variable run
, the maximum longest sequence so far in max_run
, the previous coin toss in prev
(heads
or tails
), and the total number of tosses so far in count
.
Fatal Double Down
The output from the script in Figure 2 reveals that after 21 million passes, a sequence of 23 tails in succession actually occurred. If a player had doubled the bet for the next game each time they lost, a strategy called "doubling down," they would have needed a total of 2^23 – 1=$8,388,607 of betting capital, assuming an initial bet of $1, not to go bankrupt if they had initially bet on heads, only to see 23 tails in a row with growing frustration.
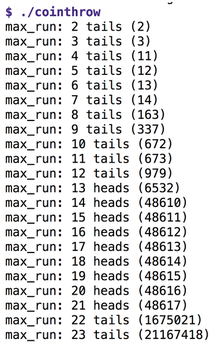
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
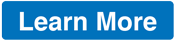
News
-
NVIDIA Released Driver for Upcoming NVIDIA 560 GPU for Linux
Not only has NVIDIA released the driver for its upcoming CPU series, it's the first release that defaults to using open-source GPU kernel modules.
-
OpenMandriva Lx 24.07 Released
If you’re into rolling release Linux distributions, OpenMandriva ROME has a new snapshot with a new kernel.
-
Kernel 6.10 Available for General Usage
Linus Torvalds has released the 6.10 kernel and it includes significant performance increases for Intel Core hybrid systems and more.
-
TUXEDO Computers Releases InfinityBook Pro 14 Gen9 Laptop
Sporting either AMD or Intel CPUs, the TUXEDO InfinityBook Pro 14 is an extremely compact, lightweight, sturdy powerhouse.
-
Google Extends Support for Linux Kernels Used for Android
Because the LTS Linux kernel releases are so important to Android, Google has decided to extend the support period beyond that offered by the kernel development team.
-
Linux Mint 22 Stable Delayed
If you're anxious about getting your hands on the stable release of Linux Mint 22, it looks as if you're going to have to wait a bit longer.
-
Nitrux 3.5.1 Available for Install
The latest version of the immutable, systemd-free distribution includes an updated kernel and NVIDIA driver.
-
Debian 12.6 Released with Plenty of Bug Fixes and Updates
The sixth update to Debian "Bookworm" is all about security mitigations and making adjustments for some "serious problems."
-
Canonical Offers 12-Year LTS for Open Source Docker Images
Canonical is expanding its LTS offering to reach beyond the DEB packages with a new distro-less Docker image.
-
Plasma Desktop 6.1 Released with Several Enhancements
If you're a fan of Plasma Desktop, you should be excited about this new point release.